Preface
Cryptocurrency trading has gained immense popularity, attracting millions of traders worldwide. However, the decentralized and largely unregulated nature of cryptocurrency markets makes them a prime target for fraudulent activities. Real-time fraud detection is crucial to protect investors and maintain the integrity of these markets.
Understanding Anomalies
Anomalies are data points that deviate significantly from the norm. In the context of cryptocurrency trading, anomalies often indicate fraudulent activities, unusual trading patterns, or system malfunctions. Detecting these anomalies is essential to ensure the security and reliability of trading platforms.
The Importance of Anomaly Detection in Crypto Markets
Anomaly detection is crucial in cryptocurrency markets for several reasons. It helps prevent financial losses by identifying fraudulent activities quickly and maintains market integrity by mitigating fraud. It protects investors by preventing scams, ensures regulatory compliance, and enhances customer trust and platform credibility through effective fraud prevention.
Type | Details |
---|---|
Post-Transaction Fraud Detection |
|
Real-Time Fraud Detection |
|
Methods for Detecting Anomalies
Method | Use Case | How They Work | Examples | When to Use |
---|---|---|---|---|
Dimensionality Reduction Models | Effective for high-dimensional data where reducing dimensionality can help identify anomalies | Reduce the dimensionality of data and identify anomalies in the lower-dimensional space | Isolation Forest, Principal Component Analysis (PCA) | When dealing with high-dimensional data and when data is not labeled, such as "fraud" or "not fraud." Isolation Forest is particularly useful for real-time fraud detection in crypto trading |
Predictive Models | Suitable for applications with labeled data and where predictive accuracy is crucial | Use machine learning to predict whether a new instance is normal or anomalous based on labeled data | Logistic regression, Random Forest, LSTM (Long Short-Term Memory) | When you have a well-labeled dataset with known instances of normal and anomalous behavior, and need high accuracy |
Statistical Models | Best for datasets with known distributions and small to moderate size | Use statistical properties of the data to identify anomalies | Seasonal Decomposition of Time Series (STL), Z-score, Grubbs' test | When you have a good understanding of the data distribution and need a straightforward method for anomaly detection |
Clustering Models | Ideal for datasets where natural groupings are expected | Group data into clusters and identify outliers | k-means, DBSCAN(Density-Based Spatial Clustering) | When the data can be clustered naturally, and outliers are significantly different from normal data points |
NeuralNet Based Models | Suitable for large, complex datasets where capturing intricate patterns is necessary | Use neural networks to learn complex data representations and identify anomalies | Autoencoders, GANs (Generative Adversarial Networks) | When dealing with highly complex data where traditional methods fall short, and computational resources are available for training deep learning models |
Isolation Forest for Crypto Trading Fraud Detection
Why Isolation Forest Algorithm is Ideal
The algorithm works by isolating anomalies using decision trees based on random attributes. This random splitting creates shorter paths for anomalies because:
Unlabeled Data
Cryptocurrency trading often doesn't have labeled data to identify fraudulent and non-fraudulent transactions. The Isolation Forest algorithm, which is an unsupervised learning method, works well in situations with limited labeled data.
Anomaly Detection Focus
Cryptocurrency markets have many transactions but only a few are fraudulent. The Isolation Forest algorithm efficiently detects these rare anomalies by isolating them quickly, making it ideal for spotting unusual patterns that indicate fraud.
Efficiency
Cryptocurrency markets produce large amounts of complex data. Isolation Forest's computational efficiency allows it to scale effectively, enabling real-time fraud detection without significant delays.
Implementing Isolation Forest Algorithm for Real-Time Detection
Let's implement a real-time fraud detection system for cryptocurrency trading using Go. We will fetch price data from the different sources, for instance Binance, CoinGecko, builds an isolation forest for anomaly detection, and reports detected anomalies along with statistics.
How Isolation Forest Algorithm Works
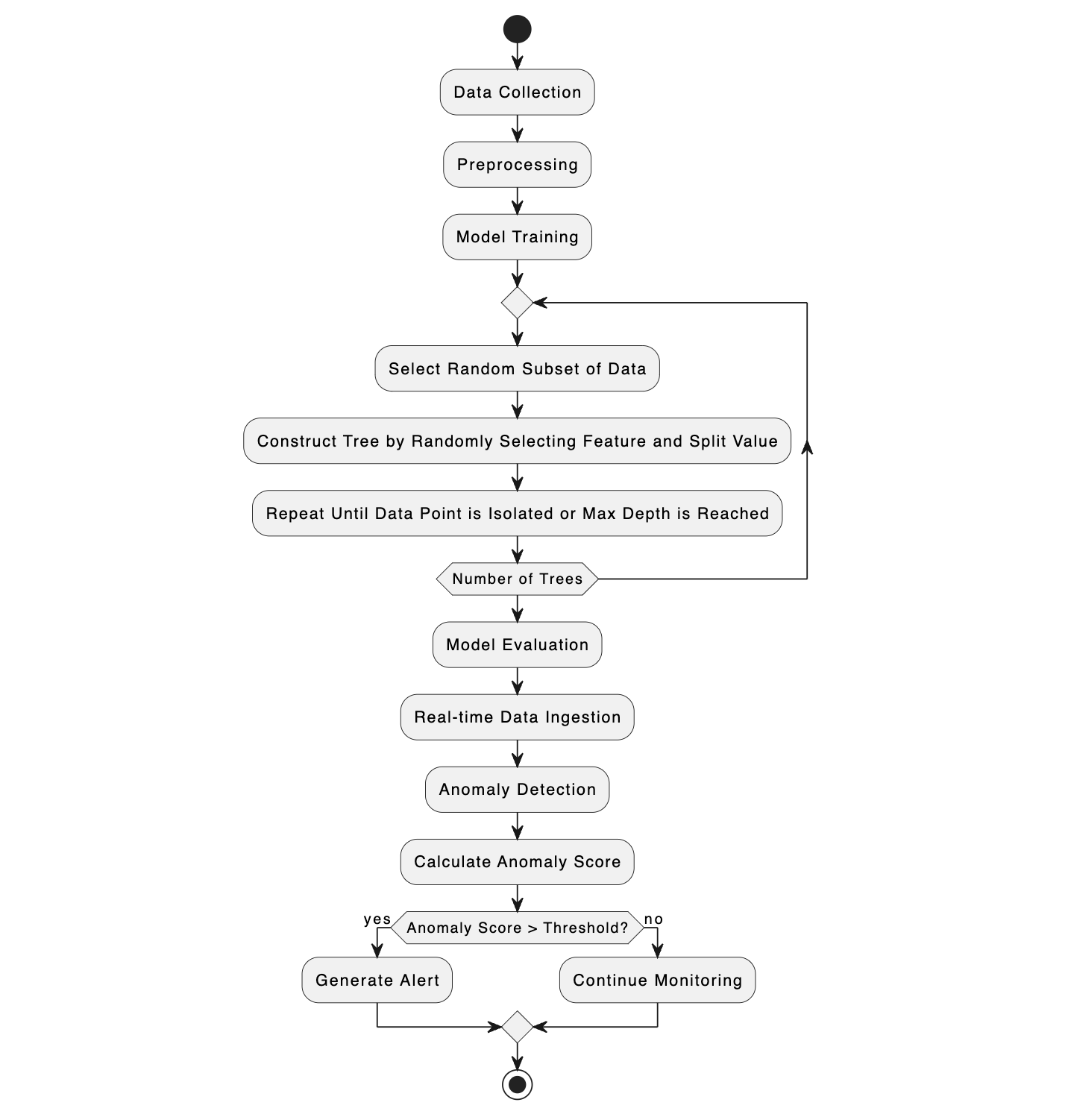
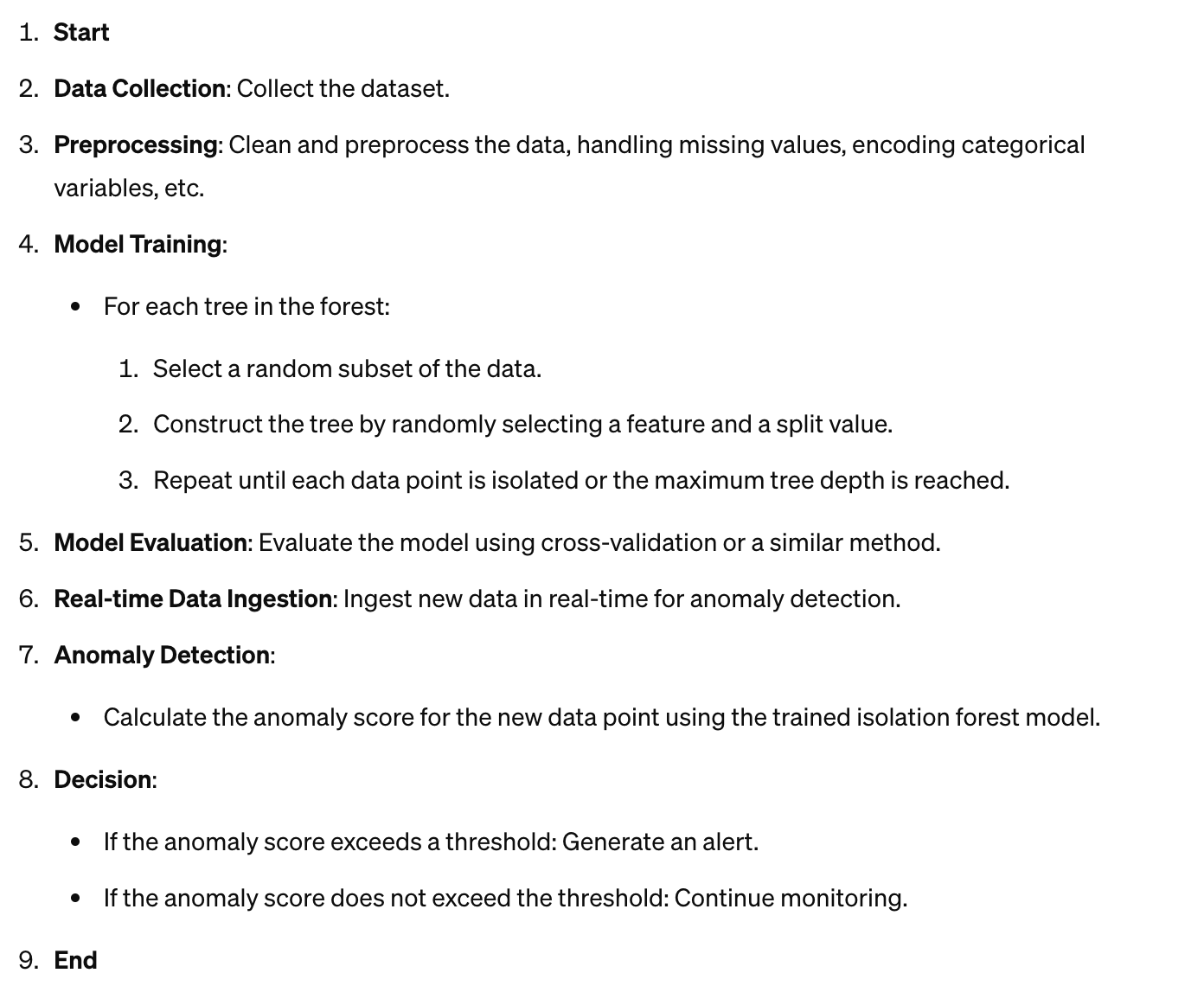
Features
- Enabled Sources: Perform operations based on enabled sources.
- Real-time Data Fetching: Continuously fetches enabled source cryptocurrency price data, e.g., Binance, CoinGecko.
- Isolation Forest: Implements an isolation forest for anomaly detection.
- Anomaly Detection: Detects anomalies in the price data and reports them.
- Statistics Reporting with Alert: Provides statistics on the total number of items and anomalies detected.
Real-Time Detection using Isolation Forest Algorithm in Golang
import (
"github.com/mdshahjahanmiah/fraud-detection/pkg/config"
"github.com/mdshahjahanmiah/fraud-detection/pkg/source"
"log/slog"
"strings"
)
func main() {
// Load configuration settings
conf, err := config.Load()
if err != nil {
slog.Error("error", "err", err)
return
}
// Channels for error handling, completion signal, anomaly detection, and statistics
errCh := make(chan error)
doneCh := make(chan struct{})
anomalyChan := make(chan string)
statsChan := make(chan string)
// Parse and initialize enabled data sources from configuration
enabledSources := strings.Split(conf.EnabledSources, ";")
for _, src := range enabledSources {
switch strings.TrimSpace(src) {
case "binance":
binanceSource := source.NewBinanceSource(conf)
go binanceSource.Start(errCh, doneCh, anomalyChan, statsChan)
case "coingecko":
coinGeckoSource := source.NewCoinGeckoSource(conf)
go coinGeckoSource.Start(errCh, doneCh, anomalyChan, statsChan)
default:
slog.Warn("unknown source", "source", src)
}
}
// Main loop to handle messages from channels
for {
select {
case err := <-errCh:
// Log any errors received
slog.Error("error", "err", err)
case anomaly := <-anomalyChan:
// Log any anomalies detected
slog.Warn("found anomalies", "anomaly", anomaly)
case stats := <-statsChan:
// Log statistics about anomalies
slog.Info("anomalies statistics", "stats", stats)
case <-doneCh:
slog.Info("processing completed")
return
}
}
}
For the full code, please visit GitHub repository, Real-Time Fraud Detection in Cryptocurrency Trading
Conclusion
Isolation Forest is a powerful dimensionality reduction model for anomaly detection, particularly effective in identifying outliers in high-dimensional datasets without the need for labeled training data. Its efficiency and unsupervised nature make it an ideal choice for real-time fraud detection in cryptocurrency trading, where data is abundant and anomalies are rare but significant
NOTE: I'm constantly delighted to receive feedback. Whether you spot an error, have a suggestion for improvement, or just want to share your thoughts, please don't hesitate to comment/reach out. I truly value connecting with readers!